React interviews questions and answers with code
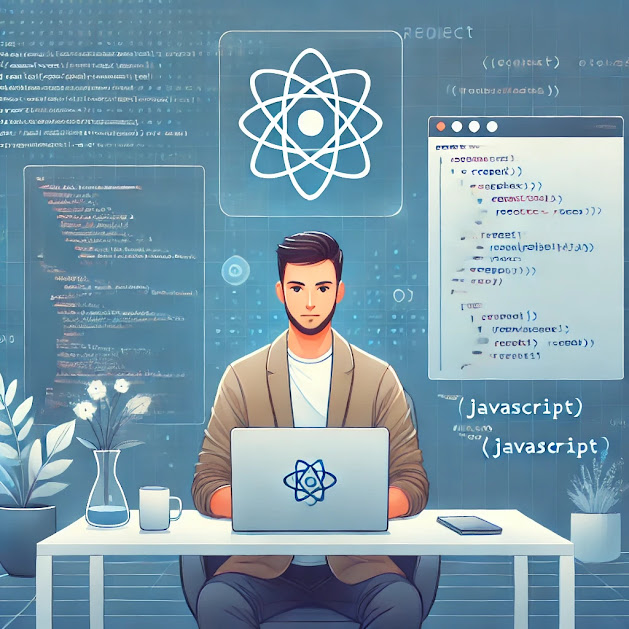
React Interview Preparation: Best Questions, Best Answers, and Sample Code 1. What are the differences between functional and class components in React? Answer : Functional components are simpler and mainly focus on rendering UI, while class components provide more powerful features like state management and lifecycle methods. In recent versions of React, hooks ( useState , useEffect ) enable functional components to use state and lifecycle features without needing to be classes. // Functional Component function FunctionalComponent(props) { const [count, setCount] = useState(0); return ( <div> <p>Count: {count}</p> <button onClick={() => setCount(count + 1)}>Increment</button> </div> ); } // Class Component class ClassComponent extends React.Component { ...