Angular interview questions
Key Concepts for Interviews
» RxJS: Master reactive programming with Observables for handling asynchronous data streams.
» Modules: Organize your app's functionality with feature-based modules.
» Routing: Navigate seamlessly between views using Angular's powerful routing system.
» Dependency Injection: Enhance flexibility by managing service dependencies dynamically.
» Angular Universal: Boost SEO and performance with server-side rendering (SSR).
» AOT Compilation: Optimize app performance by compiling templates during the build phase.
» Custom Pipes: Transform data in templates with built-in or user-defined pipes.
interview questions ⇲
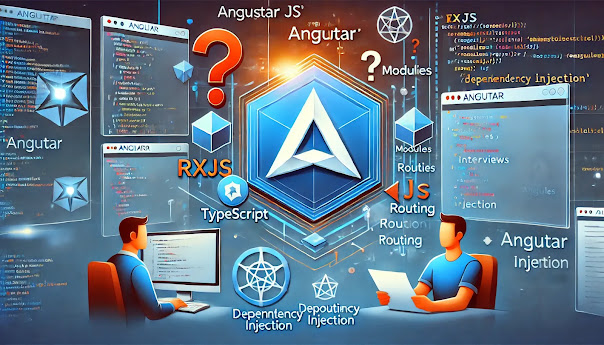
1. What are Angular Directives?
Question: Explain Angular directives. Can you give examples?
Answer: Angular directives are used to extend the HTML by adding custom behavior to elements. There are three types:
- Components: A directive with a template.
- Structural Directives: Change the DOM structure (
*ngIf
,*ngFor
). - Attribute Directives: Change the appearance or behavior of an element (
[ngStyle]
,[ngClass]
).
Example Code:
// Custom attribute directive
import { Directive, ElementRef, Renderer2, HostListener } from '@angular/core';
@Directive({
selector: '[appHighlight]'
})
export class HighlightDirective {
constructor(private el: ElementRef, private renderer: Renderer2) {}
@HostListener('mouseenter') onMouseEnter() {
this.renderer.setStyle(this.el.nativeElement, 'backgroundColor', 'yellow');
}
@HostListener('mouseleave') onMouseLeave() {
this.renderer.removeStyle(this.el.nativeElement, 'backgroundColor');
}
}
2. Explain the Lifecycle Hooks in Angular
Question: What are Angular lifecycle hooks, and why are they used?
Answer: Angular provides lifecycle hooks to tap into the different stages of a component or directive. Common hooks are:
ngOnInit
: Called after the component is initialized.ngOnChanges
: Invoked when input properties change.ngOnDestroy
: Called before the component is destroyed.
Example Code:
import { Component, OnInit, OnDestroy } from '@angular/core';
@Component({
selector: 'app-demo',
template: `<p>Demo Component Loaded</p>`
})
export class DemoComponent implements OnInit, OnDestroy {
constructor() {
console.log('Constructor called');
}
ngOnInit() {
console.log('ngOnInit called');
}
ngOnDestroy() {
console.log('ngOnDestroy called');
}
}
3. How Does Angular Handle Dependency Injection?
Question: What is dependency injection in Angular, and how is it implemented?
Answer: Dependency Injection (DI) is a design pattern where objects are provided their dependencies rather than creating them. Angular uses DI to manage services.
Example Code:
// Service
import { Injectable } from '@angular/core';
@Injectable({
providedIn: 'root',
})
export class DataService {
getData() {
return ['Angular', 'React', 'Vue'];
}
}
// Component
import { Component, OnInit } from '@angular/core';
import { DataService } from './data.service';
@Component({
selector: 'app-data',
template: `<ul><li *ngFor="let item of data">{{ item }}</li></ul>`
})
export class DataComponent implements OnInit {
data: string[] = [];
constructor(private dataService: DataService) {}
ngOnInit() {
this.data = this.dataService.getData();
}
}
4. What is Change Detection in Angular?
Question: Explain Angular's change detection mechanism.
Answer: Angular's change detection checks the state of the application and updates the DOM whenever data changes. It uses a zone.js library to intercept asynchronous operations and a digest cycle to compare the old and new values.
Example Code:
import { Component } from '@angular/core';
@Component({
selector: 'app-counter',
template: `
<button (click)="increment()">Increment</button>
<p>Counter: {{ counter }}</p>
`
})
export class CounterComponent {
counter = 0;
increment() {
this.counter++;
}
}
5. What is Lazy Loading in Angular?
Question: How do you implement lazy loading in Angular?
Answer: Lazy loading is a technique to load only the required feature modules on demand to optimize performance.
Example Code:
1. Create a feature module:
//bash
ng generate module feature --route feature --module app.module
2. Angular sets up lazy loading:
6. What is an Angular Form? Difference Between Template-Driven and Reactive Forms
Question: Explain Angular forms and the difference between template-driven and reactive forms.
Answer:
- Template-driven Forms: Defined in HTML using directives like
ngModel
. - Reactive Forms: Defined in the component class using
FormControl
andFormGroup
.
Example Code (Reactive Form):
import { Component } from '@angular/core';
import { FormGroup, FormControl, Validators } from '@angular/forms';
@Component({
selector: 'app-reactive-form',
template: `
<form [formGroup]="loginForm" (ngSubmit)="onSubmit()">
<input formControlName="email" placeholder="Email" />
<input formControlName="password" placeholder="Password" type="password" />
<button type="submit" [disabled]="!loginForm.valid">Submit</button>
</form>
`
})
export class ReactiveFormComponent {
loginForm = new FormGroup({
email: new FormControl('', [Validators.required, Validators.email]),
password: new FormControl('', Validators.required)
});
onSubmit() {
console.log(this.loginForm.value);
}
}
7. How to Optimize Angular Applications?
Question: Name techniques to optimize Angular applications.
Answer:
- Use
OnPush
Change Detection:
changeDetection: ChangeDetectionStrategy.OnPush })
({ - Lazy Load Modules.
- Use Angular CLI Production Build:
- Optimize Observables with
takeUntil
to avoid memory leaks. - Enable Ahead-of-Time (AOT) Compilation.
8. What is the Purpose of Angular Pipes?
Question: Explain Angular pipes and give examples of custom pipes.
Answer: Pipes in Angular are used to transform data in the template. Common built-in pipes include date
, uppercase
, and currency
. You can also create custom pipes for specific transformations.
Example Code:
// Custom Pipe
import { Pipe, PipeTransform } from '@angular/core';
@Pipe({
name: 'reverse'
})
export class ReversePipe implements PipeTransform {
transform(value: string): string {
return value.split('').reverse().join('');
}
}
// Component
@Component({
selector: 'app-pipe-demo',
template: `<p>{{ 'Angular' | reverse }}</p>`
})
export class PipeDemoComponent {}
9. What is the Role of NgZone
in Angular?
Question: What is NgZone
, and how is it used?
Answer: NgZone
allows Angular to execute tasks outside of its default zone for improved performance, especially when handling heavy operations.
Example Code:
import { Component, NgZone } from '@angular/core';
@Component({
selector: 'app-zone-demo',
template: `<p>{{ progress }}%</p>`
})
export class ZoneDemoComponent {
progress = 0;
constructor(private ngZone: NgZone) {}
simulateHeavyTask() {
this.ngZone.runOutsideAngular(() => {
this.progress = 0;
const interval = setInterval(() => {
this.progress += 10;
if (this.progress === 100) {
clearInterval(interval);
this.ngZone.run(() => console.log('Task Completed!'));
}
}, 100);
});
}
}
10. What Are Guards in Angular?
Question: What are Angular guards, and how do you use them?
Answer: Guards are used to control access to routes in Angular. There are different types of guards:
CanActivate
: Controls if a route can be activated.CanDeactivate
: Checks if a user can leave a route.
Example Code (CanActivate):
// Auth Guard
import { Injectable } from '@angular/core';
import { CanActivate, Router } from '@angular/router';
@Injectable({
providedIn: 'root'
})
export class AuthGuard implements CanActivate {
constructor(private router: Router) {}
canActivate(): boolean {
const isLoggedIn = false; // Simulated check
if (!isLoggedIn) {
this.router.navigate(['/login']);
return false;
}
return true;
}
}
// Route Configuration
const routes: Routes = [
{ path: 'dashboard', component: DashboardComponent, canActivate: [AuthGuard] }
];
11. What is RxJS, and How is it Used in Angular?
Question: Explain RxJS and give an example of using Observables in Angular.
Answer: RxJS (Reactive Extensions for JavaScript) is a library for reactive programming using Observables, which are used in Angular for handling asynchronous operations like HTTP requests.
Example Code:
import { Component, OnInit } from '@angular/core';
import { Observable } from 'rxjs';
@Component({
selector: 'app-observable-demo',
template: `<p>{{ message }}</p>`
})
export class ObservableDemoComponent implements OnInit {
message: string = '';
ngOnInit() {
const observable = new Observable<string>((observer) => {
observer.next('Hello');
setTimeout(() => observer.next('World!'), 1000);
setTimeout(() => observer.complete(), 2000);
});
observable.subscribe({
next: (msg) => (this.message += msg + ' '),
complete: () => console.log('Observable completed!')
});
}
}
12. What is Angular Universal?
Question: What is Angular Universal, and why is it used?
Answer: Angular Universal is a tool for server-side rendering (SSR) of Angular applications. It improves SEO, performance, and initial load times by rendering HTML on the server.
Example Steps:
1. Install Angular Universal:
13. What Are Subject and BehaviorSubject in Angular?
Question: Explain the difference between Subject and BehaviorSubject in RxJS.
Answer:
- Subject: Emits values only to new subscribers from the time of subscription.
- BehaviorSubject: Stores the latest value and emits it immediately to new subscribers.
Example Code:
import { Component, OnInit } from '@angular/core';
import { BehaviorSubject } from 'rxjs';
@Component({
selector: 'app-behavior-subject-demo',
template: `<p>Value: {{ value }}</p>`
})
export class BehaviorSubjectDemoComponent implements OnInit {
private subject = new BehaviorSubject<number>(0);
value: number = 0;
ngOnInit() {
this.subject.subscribe((val) => (this.value = val));
this.subject.next(1); // Updates value to 1
}
}
14. How to Handle HTTP Interceptors in Angular?
Question: What are HTTP interceptors, and how are they implemented?
Answer: HTTP interceptors modify or handle HTTP requests and responses globally.
Example Code:
import { Injectable } from '@angular/core';
import { HttpInterceptor, HttpRequest, HttpHandler } from '@angular/common/http';
@Injectable()
export class AuthInterceptor implements HttpInterceptor {
intercept(req: HttpRequest<any>, next: HttpHandler) {
const clonedRequest = req.clone({
setHeaders: { Authorization: `Bearer fake-token` }
});
return next.handle(clonedRequest);
}
}
// App Module
import { HTTP_INTERCEPTORS } from '@angular/common/http';
@NgModule({
providers: [
{ provide: HTTP_INTERCEPTORS, useClass: AuthInterceptor, multi: true }
]
})
export class AppModule {}
15. How Does Angular Handle Error Handling?
Question: How can errors be handled globally in Angular?
Answer: Errors can be handled globally using HttpInterceptor
or a global ErrorHandler
.
Example Code (Global ErrorHandler):
import { ErrorHandler, Injectable } from '@angular/core';
@Injectable()
export class GlobalErrorHandler implements ErrorHandler {
handleError(error: any): void {
console.error('Global Error:', error);
}
}
// App Module
@NgModule({
providers: [
{ provide: ErrorHandler, useClass: GlobalErrorHandler }
]
})
export class AppModule {}
16. What is Ahead-of-Time (AOT) Compilation in Angular?
Question: Explain Ahead-of-Time (AOT) compilation in Angular.
Answer: Ahead-of-Time (AOT) compilation converts Angular HTML and TypeScript code into efficient JavaScript code during the build process. This improves performance and reduces load time by avoiding runtime template compilation.
Advantages of AOT:
- Faster rendering.
- Smaller application size.
- Detects template errors at build time.
How to Enable AOT?
Run the Angular build command with AOT enabled:
//bash
ng build --prod
17. What Are Dynamic Components in Angular?
Question: How do you create and load dynamic components in Angular?
Answer: Dynamic components are created and loaded at runtime using Angular’s ComponentFactoryResolver
and ViewContainerRef
.
Example Code:
import { Component, ComponentFactoryResolver, ViewChild, ViewContainerRef } from '@angular/core';
// Dynamic Component
@Component({
selector: 'app-dynamic',
template: `<p>I am a dynamic component!</p>`
})
export class DynamicComponent {}
// Parent Component
@Component({
selector: 'app-parent',
template: `<button (click)="loadComponent()">Load Dynamic Component</button>
<ng-template #container></ng-template>`
})
export class ParentComponent {
@ViewChild('container', { read: ViewContainerRef }) container!: ViewContainerRef;
constructor(private resolver: ComponentFactoryResolver) {}
loadComponent() {
const factory = this.resolver.resolveComponentFactory(DynamicComponent);
this.container.clear();
this.container.createComponent(factory);
}
}
18. What is the Difference Between ViewChild
and ContentChild
?
Question: Explain the difference between ViewChild
and ContentChild
in Angular.
Answer:
ViewChild
: Access elements or components inside the view (template) of a component.ContentChild
: Access projected content passed to a component via<ng-content>
.
Example Code:
// Child Component
@Component({
selector: 'app-child',
template: `<p>Child Component</p>`
})
export class ChildComponent {}
// Parent Component
@Component({
selector: 'app-parent',
template: `
<app-child #childView></app-child>
<ng-content></ng-content>
`
})
export class ParentComponent {
@ViewChild('childView') child!: ChildComponent;
}
19. How Do You Create a Custom Validator in Angular?
Question: How can you implement a custom form validator?
Answer:
A custom validator is a function that checks the validity of a form control.
Example Code:
import { AbstractControl, ValidationErrors, ValidatorFn } from '@angular/forms';
// Custom Validator Function
export function forbiddenNameValidator(forbiddenName: string): ValidatorFn {
return (control: AbstractControl): ValidationErrors | null => {
const forbidden = control.value === forbiddenName;
return forbidden ? { forbiddenName: { value: control.value } } : null;
};
}
// Applying the Validator in a Form
@Component({
selector: 'app-custom-validator',
template: `
<form [formGroup]="form">
<input formControlName="username" placeholder="Username" />
<div *ngIf="form.get('username')?.hasError('forbiddenName')">
Username is forbidden!
</div>
</form>
`
})
export class CustomValidatorComponent {
form = new FormGroup({
username: new FormControl('', forbiddenNameValidator('admin'))
});
}
20. What is a Singleton Service in Angular?
Question: What is a singleton service, and how do you create one?
Answer: A singleton service is a service that has a single instance shared across the application. To make a service singleton, provide it at the root level.
Example Code:
@Injectable({
providedIn: 'root' // Singleton service
})
export class SingletonService {
private counter = 0;
increment() {
this.counter++;
return this.counter;
}
}
// Using the Service
@Component({
selector: 'app-counter',
template: `<button (click)="increment()">Increment</button> {{ count }}`
})
export class CounterComponent {
count = 0;
constructor(private singletonService: SingletonService) {}
increment() {
this.count = this.singletonService.increment();
}
}
21. What Are Angular Animations?
Question: How do you implement animations in Angular?
Answer: Angular provides the @angular/animations
module for creating animations.
Example Code:
import { Component } from '@angular/core';
import { trigger, state, style, transition, animate } from '@angular/animations';
@Component({
selector: 'app-animation',
template: `
<div [@fadeInOut]="isVisible ? 'visible' : 'hidden'" class="box"></div>
<button (click)="toggle()">Toggle Animation</button>
`,
styles: [`.box { width: 100px; height: 100px; background: blue; }`],
animations: [
trigger('fadeInOut', [
state('visible', style({ opacity: 1 })),
state('hidden', style({ opacity: 0 })),
transition('visible <=> hidden', [animate('500ms ease-in-out')])
])
]
})
export class AnimationComponent {
isVisible = true;
toggle() {
this.isVisible = !this.isVisible;
}
}
22. What Are Angular Modules? Why Are They Important?
Question: Explain Angular modules and their significance.
Answer: An Angular module (@NgModule
) is a container for components, directives, pipes, and services. It organizes the application into cohesive blocks.
Key Parts of an Angular Module:
declarations
: Components, directives, and pipes.imports
: Other modules.providers
: Services.bootstrap
: Root component to bootstrap.
Example Code:
@NgModule({
declarations: [AppComponent],
imports: [BrowserModule, FormsModule],
providers: [],
bootstrap: [AppComponent]
})
export class AppModule {}
23. What is the Purpose of RouterModule.forRoot()
?
Question: Explain the role of RouterModule.forRoot()
in Angular routing.
Answer: RouterModule.forRoot()
configures the root-level routes of an Angular application. It is called only once in the root module.
Example Code:
const routes: Routes = [
{ path: '', component: HomeComponent },
{ path: 'about', component: AboutComponent }
];
@NgModule({
imports: [RouterModule.forRoot(routes)],
exports: [RouterModule]
})
export class AppRoutingModule {}
Frequently Asked Questions (FAQs)
{{ name }}
Did you find this article valuable?
Support
by becoming a sponsor.Share
# Tags