Implementing Microservices with Python
A guide on building and deploying microservices using Python, including best practices, tools, and frameworks like Flask and FastAPI.
Implementing Microservices with Python
Microservices architecture has become a popular approach for building scalable and maintainable applications. It involves breaking down a large application into smaller, independent services that can be developed, deployed, and scaled independently. Python, with its rich ecosystem of libraries and frameworks, is an excellent choice for implementing microservices. This guide will explore how to build and deploy microservices using Python, with a focus on Flask and FastAPI.
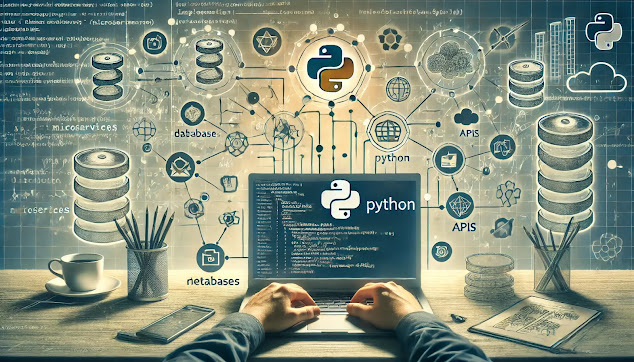
Why Microservices?
Microservices offer several advantages over monolithic architectures:
Scalability: Each service can be scaled independently based on its load.
Maintainability: Smaller codebases are easier to understand, test, and maintain.
Deployment: Services can be deployed independently, allowing for more frequent and safer deployments.
Technology Diversity: Different services can use different technologies that best suit their needs.
Best Practices for Microservices
Single Responsibility Principle: Each microservice should have a single responsibility and perform a specific function.
Decentralized Data Management: Each service should manage its own database to avoid tight coupling.
Inter-Service Communication: Use lightweight protocols like HTTP/REST or messaging queues like RabbitMQ or Kafka for communication.
Service Discovery: Implement service discovery to dynamically locate services.
API Gateway: Use an API gateway to handle common concerns like authentication, logging, and rate limiting.
Monitoring and Logging: Implement comprehensive monitoring and logging to track the health and performance of services.
Automated Testing: Ensure each service has a robust suite of automated tests.
Tools and Frameworks
Flask
Flask is a micro web framework for Python, making it a popular choice for building microservices due to its simplicity and flexibility.
Example: Creating a simple microservice with Flask
python
from flask import Flask, jsonify, request
app = Flask(__name__)
@app.route('/hello', methods=['GET'])
def hello():
return jsonify(message='Hello, World!')
@app.route('/add', methods=['POST'])
def add():
data = request.get_json()
result = data['a'] + data['b']
return jsonify(result=result)
if __name__ == '__main__':
app.run(debug=True, host='0.0.0.0', port=5000)
FastAPI
FastAPI is a modern, fast (high-performance), web framework for building APIs with Python 3.6+ based on standard Python type hints. It's designed to be easy to use and highly performant.
Example: Creating a simple microservice with FastAPI
python
from fastapi import FastAPI
from pydantic import BaseModel
app = FastAPI()
class AddRequest(BaseModel):
a: int
b: int
@app.get('/hello')
def hello():
return {'message': 'Hello, World!'}
@app.post('/add')
def add(request: AddRequest):
result = request.a + request.b
return {'result': result}
if __name__ == '__main__':
import uvicorn
uvicorn.run(app, host='0.0.0.0', port=8000)
Deploying Microservices
Deployment of microservices can be handled using various containerization and orchestration tools.
Docker
Docker is a platform for developing, shipping, and running applications inside containers. Containers package the application and its dependencies, ensuring consistency across environments.
Example: Dockerfile for a Flask microservice
FROM python:3.9-slim
WORKDIR /app
COPY requirements.txt requirements.txt
RUN pip install -r requirements.txt
COPY . .
CMD ["python", "app.py"]
Example: Dockerfile for a FastAPI microservice
FROM python:3.9-slim
WORKDIR /app
COPY requirements.txt requirements.txt
RUN pip install -r requirements.txt
COPY . .
CMD ["uvicorn", "main:app", "--host", "0.0.0.0", "--port", "8000"]
Kubernetes
Kubernetes is an open-source system for automating the deployment, scaling, and management of containerized applications.
Example: Kubernetes Deployment for a Flask microservice
yaml
apiVersion: apps/v1
kind: Deployment
metadata:
name: flask-microservice
spec:
replicas: 3
selector:
matchLabels:
app: flask-microservice
template:
metadata:
labels:
app: flask-microservice
spec:
containers:
- name: flask-microservice
image: your-docker-image
ports:
- containerPort: 5000
Additional Considerations
Security: Ensure secure communication between microservices using HTTPS and implement robust authentication and authorization mechanisms.
Fault Tolerance: Implement circuit breakers and retries to handle transient failures gracefully.
Data Consistency: Consider using eventual consistency and patterns like Saga for managing transactions across multiple services.
Configuration Management: Use tools like Consul or etcd for managing configuration centrally.
Service Mesh: Consider using a service mesh like Istio for managing microservices networking, security, and observability.
Monitoring and Logging Tools
Prometheus: Open-source monitoring and alerting toolkit.
Grafana: Open-source platform for monitoring and observability.
ELK Stack (Elasticsearch, Logstash, Kibana): Powerful log management and analysis tool.
Automated Testing Tools
PyTest: A mature full-featured Python testing tool.
Mock: Allows you to replace parts of your system under test and make assertions about how they have been used.
Postman: For testing APIs.
CI/CD Pipelines
Continuous Integration and Continuous Deployment (CI/CD) pipelines are essential for automating the deployment process.
GitHub Actions: Automate workflows directly from your GitHub repository.
GitLab CI/CD: Full CI/CD tool integrated with GitLab.
Jenkins: Open-source automation server.
Example: Building a CI/CD Pipeline for a Flask Microservice
GitHub Actions Workflow Configuration
yaml
name: CI/CD Pipeline
on:
push:
branches:
- main
jobs:
build:
runs-on: ubuntu-latest
steps:
- name: Checkout code
uses: actions/checkout@v2
- name: Set up Python
uses: actions/setup-python@v2
with:
python-version: 3.9
- name: Install dependencies
run: |
python -m pip install --upgrade pip
pip install -r requirements.txt
- name: Run tests
run: |
pytest
- name: Build Docker image
run: |
docker build . -t your-docker-image
- name: Push Docker image
run: |
echo "${{ secrets.DOCKER_PASSWORD }}" | docker login
-u "$ {{ secrets.DOCKER_USERNAME }}"--password-stdin
docker push your-docker-image
Deploying to Kubernetes
After building and pushing the Docker image, the next step is to deploy it to Kubernetes. You can use tools like kubectl or Helm for managing your Kubernetes resources.
Example: Kubernetes Deployment Script
yaml
apiVersion: apps/v1
kind: Deployment
metadata:
name: flask-microservice
spec:
replicas: 3
selector:
matchLabels:
app: flask-microservice
template:
metadata:
labels:
app: flask-microservice
spec:
containers:
- name: flask-microservice
image: your-docker-image
ports:
- containerPort: 5000
---
apiVersion: v1
kind: Service
metadata:
name: flask-microservice
spec:
type: LoadBalancer
ports:
- port: 80
targetPort: 5000
selector:
app: flask-microservice
Resources for Further Learning
Books:
- "Building Microservices" by Sam Newman
- "Microservices with Docker, Flask, and React" by Nigel George
Online Courses:
- Coursera: "Microservices Specialization" by University of California, Berkeley
- Udemy: "Microservices with Python" by Jose Salvatierra
Case Studies and Real-World Examples
Understanding how leading companies implement microservices can provide valuable insights. Here are a few case studies that demonstrate the use of microservices architecture:
Case Study 1: Netflix
Netflix is one of the pioneers of microservices architecture. They transitioned from a monolithic application to a cloud-based, microservices architecture to handle their vast user base and the large volume of streaming content.
Key Points:
Scalability: Each microservice can be scaled independently to meet the demand.
Resilience: By breaking down the application, Netflix can isolate failures and prevent them from affecting the entire system.
Technology Diversity: Services can be built using the best technology suited for the task.
Case Study 2: Uber
Uber moved from a monolithic architecture to microservices to accommodate their rapid growth and the need for high availability and fault tolerance.
Key Points:
Independent Deployment: Services can be deployed independently without affecting the entire system.
Improved Development Velocity: Teams can develop, test, and deploy their services independently.
Resilience and Fault Tolerance: Microservices allow for better fault isolation and quicker recovery from failures.
Challenges and Solutions
Implementing microservices comes with its own set of challenges. Here are some common challenges and how to address them:
Complexity in Management:
Solution: Use orchestration tools like Kubernetes to manage containerized services efficiently.
Inter-Service Communication:
Solution: Implement lightweight communication protocols (e.g., HTTP/REST, gRPC) and use message brokers (e.g., RabbitMQ, Kafka) for asynchronous communication.
Data Consistency:
Solution: Adopt eventual consistency models and use patterns like Saga for managing distributed transactions.
Monitoring and Debugging:
Solution: Implement centralized logging (e.g., ELK Stack) and monitoring solutions (e.g., Prometheus, Grafana) to gain visibility into the health of services.
Security:
Solution: Use HTTPS for secure communication, implement robust authentication and authorization mechanisms, and follow security best practices.
Future Trends in Microservices
As the technology landscape evolves, so do the approaches and tools for implementing microservices. Here are some future trends to watch:
Service Meshes: Tools like Istio and Linkerd are gaining popularity for managing the communication between microservices, providing features like load balancing, service discovery, and observability.
Serverless Architectures: Combining microservices with serverless computing (e.g., AWS Lambda, Azure Functions) can further simplify deployment and scaling by eliminating server management.
Edge Computing: Deploying microservices closer to the data source (e.g., IoT devices) to reduce latency and improve performance.
AI and Machine Learning: Integrating AI/ML models into microservices to enhance functionalities and provide intelligent features.
Additional Resources and References
To deepen your understanding of microservices with Python, here are some additional resources and references:
Online Courses and Tutorials
Coursera:
- "Microservices Specialization" by the University of California, Berkeley
- "Cloud Computing Specialization" by the University of Illinois at Urbana-Champaign
Udemy:
- "Microservices with Python: Build, Deploy, and Scale" by Jose Salvatierra
- "Master Microservices with Spring Boot and Spring Cloud" by in28Minutes Official (for a comparative study with Java-based microservices)
Pluralsight:
- "Building Microservices" by Richard Seroter
- "Microservices: The Big Picture" by Kevin Crawley
Books
- "Building Microservices" by Sam Newman
- "Microservices Patterns" by Chris Richardson
- "Designing Data-Intensive Applications" by Martin Kleppmann
- "Microservices with Docker, Flask, and React" by Nigel George
Documentation and Blogs
- Flask Documentation: Flask Official Documentation
- FastAPI Documentation: FastAPI Official Documentation
- Docker Documentation: Docker Official Documentation
- Kubernetes Documentation: Kubernetes Official Documentation
Tools and Platforms
Service Meshes:
- Istio: Istio Documentation
- Linkerd: Linkerd Documentation
Serverless Platforms:
- AWS Lambda: AWS Lambda Documentation
- Azure Functions: Azure Functions Documentation
Monitoring and Logging:
- Prometheus: Prometheus Documentation
- Grafana: Grafana Documentation
- ELK Stack: Elastic Stack Documentation
Messaging and Communication:
- RabbitMQ: RabbitMQ Documentation
- Apache Kafka: Kafka Documentation
Community and Support
Engaging with the community and seeking support when needed is crucial for staying up-to-date and resolving challenges. Here are some platforms and communities:
Stack Overflow: A valuable resource for asking questions and finding answers related to microservices, Python, Flask, FastAPI, and related technologies.
GitHub: Explore repositories, contribute to open-source projects, and collaborate with other developers.
Reddit: Subreddits like r/Python, r/microservices, and r/devops provide discussions, news, and insights from the developer community.
Slack and Discord Communities: Join channels related to Python, microservices, and DevOps to connect with like-minded professionals.
Practical Steps to Get Started
Set Up Your Development Environment: Ensure you have Python installed, along with necessary tools like Docker, kubectl, and a code editor (e.g., VS Code).
Choose Your Framework: Decide whether to use Flask or FastAPI based on your project requirements.
Create Your First Microservice: Start by building a simple microservice, focusing on a single functionality.
Containerize Your Service: Write a Dockerfile to containerize your microservice.
Deploy Your Service Locally: Use Docker Compose to run your microservice locally and test inter-service communication.
Set Up CI/CD Pipeline: Automate your build and deployment process using GitHub Actions or another CI/CD tool.
Deploy to Kubernetes: Deploy your microservice to a Kubernetes cluster and scale it as needed.
Implement Monitoring and Logging: Set up Prometheus and Grafana for monitoring and use the ELK stack for centralized logging.
Iterate and Improve: Continuously improve your microservice by following best practices, optimizing performance, and ensuring security.
Frequently Asked Questions (FAQs)
Flask: Flask is a micro web framework for Python that is simple to use and highly flexible. It is well-suited for small to medium-sized applications and provides a straightforward way to build microservices.
FastAPI: FastAPI is a modern, fast (high-performance) web framework for building APIs with Python 3.6+ based on standard Python type hints. It is designed for high performance, ease of use, and offers automatic generation of interactive API documentation (Swagger UI).
FastAPI: FastAPI is a modern, fast (high-performance) web framework for building APIs with Python 3.6+ based on standard Python type hints. It is designed for high performance, ease of use, and offers automatic generation of interactive API documentation (Swagger UI).
Consider the following factors:
Performance: FastAPI is built for high performance and can handle more requests per second compared to Flask.
Ease of Use: Flask is simpler to set up and use, making it a good choice for small projects or quick prototypes.
Type Safety: FastAPI leverages Python type hints for better code validation and automatic documentation generation, making it ideal for larger projects where type safety is crucial.
Community and Ecosystem: Flask has been around longer and has a larger community and more third-party extensions.
Performance: FastAPI is built for high performance and can handle more requests per second compared to Flask.
Ease of Use: Flask is simpler to set up and use, making it a good choice for small projects or quick prototypes.
Type Safety: FastAPI leverages Python type hints for better code validation and automatic documentation generation, making it ideal for larger projects where type safety is crucial.
Community and Ecosystem: Flask has been around longer and has a larger community and more third-party extensions.
Overcomplicating Architecture: Avoid breaking down services too granularly at the start. Start with larger services and break them down as needed.
Poor API Design: Ensure your APIs are well-designed and follow best practices for RESTful services.
Neglecting Monitoring and Logging: Implement comprehensive monitoring and logging from the beginning to quickly identify and resolve issues.
Ignoring Security: Implement robust security measures, including HTTPS, authentication, and authorization.
Insufficient Testing: Ensure each service has thorough unit, integration, and end-to-end tests.
Poor API Design: Ensure your APIs are well-designed and follow best practices for RESTful services.
Neglecting Monitoring and Logging: Implement comprehensive monitoring and logging from the beginning to quickly identify and resolve issues.
Ignoring Security: Implement robust security measures, including HTTPS, authentication, and authorization.
Insufficient Testing: Ensure each service has thorough unit, integration, and end-to-end tests.
Use centralized configuration management tools like Consul, etcd, or Spring Cloud Config to manage configuration across services. These tools provide features like dynamic configuration updates, versioning, and secure storage of sensitive information.
Use patterns like Saga and Event Sourcing to manage distributed transactions and eventual consistency. These patterns help ensure data consistency without compromising the independence and scalability of services.
Like
Share
# Tags
Share
# Tags