Variables, Data Types, and Operators in JavaScript
Variables
1. Declaration
var
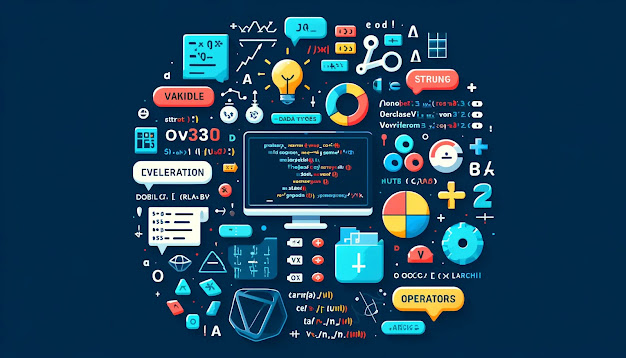
Historically, variables were declared using the var keyword. However, it has some scoping issues and is generally replaced by let and const in modern JavaScript.
javascript
var x = 10;
let
Introduced in ES6, let allows you to declare block-scoped variables. This means the variable is only available within the block it is defined.
javascript
let y = 20;
const
Also introduced in ES6, const is used to declare block-scoped variables that are read-only. Once a value is assigned to a const variable, it cannot be reassigned.
javascript
const z = 30;
2. Variable Scope
Global Scope: Variables declared outside of any function or block are in the global scope and can be accessed from anywhere in the code.
Function Scope: Variables declared within a function using var are confined to the function scope.
Block Scope: Variables declared with let
or const
within a block (denoted by {}) are confined to that block.
javascript
if (true) {
var a = 40; // global scope (if not in a function)
let b = 50; // block scope
const c = 60; // block scope
}
console.log(a); // 40
console.log(b); // Error: b is not defined
console.log(c); // Error: c is not defined
3. Variable Hoisting
JavaScript hoists variable declarations to the top of
their scope. However, only the declaration is hoisted,
not the initialization.
javascript
console.log(d); // undefined
var d = 70;
console.log(e); // Error: e is not defined
let e = 80;
4. Initialization and Assignment
Variables can be initialized when declared or assigned
a value later.
javascript
let f;
f = 90; // Assignment
5. Data Types
Variables in JavaScript can hold different data types such as:
Primitive Types: Number, String, Boolean, Null, Undefined, Symbol, and BigInt
Reference Types: Object, Array, Function, etc.
javascript
let g = 100; // Number
let h = "Hello"; // String
let i = true; // Boolean
let j = null; // Null
let k; // Undefined
let l = Symbol('id'); // Symbol
let m = 9007199254740991n; // BigInt
let n = { name: "Alice" }; // Object
let o = [1, 2, 3]; // Array
let p = function() { // Function
return "Hello, world!";
};
6. Best Practices
Prefer let and const over var for better scoping control.
Use const for variables that should not change.
Use meaningful variable names for better code readability.
Example
javascript
const pi = 3.14159; // `pi` is a constant
let radius = 10; // `radius` can change
let area = pi * radius * radius; // `area` is calculated
based on `pi` and `radius`
console.log(area); // Output: 314.159
Data Types
In JavaScript, data types are essential for understanding how values are stored and manipulated. JavaScript has several data types, which can be broadly classified into two categories: primitive data types and non-primitive (complex) data types.
Primitive Data Types
Number: Represents both integer and floating-point numbers.
Examples:
javascript
let age = 25;
let pi = 3.14;
String: Represents a sequence of characters enclosed in single quotes ('), double quotes ("), or backticks (`).
Examples:
javascript
let greeting = 'Hello, world!';
let name = "Alice";
let message = `Hello, ${name}!`;
Boolean: Represents a logical entity having two values: true and false.
Examples:
javascript
let isJavaScriptFun = true;
let isRaining = false;
Undefined: Represents a variable that has been declared but not yet assigned a value.
Example:
javascript
let a;
console.log(a); // undefined
Null: Represents the intentional absence of any object value. It is a primitive value that represents the null, empty, or non-existent reference.
Example:
javascript
let emptyValue = null;
Symbol: Represents a unique and immutable value that can be used as the key of an object property. Symbols are often used to avoid property name collisions.
Example:
javascript
let sym = Symbol('description');
console.log(sym); // Symbol(description)
BigInt: Represents whole numbers larger than the largest number JavaScript can reliably represent with the Number primitive (2^53 - 1). BigInt is created by appending n to the end of an integer.
Example:
javascript
let bigNumber =
1234567890123456789012345678901234567890n;
Non-Primitive Data Types
Object: Represents a collection of properties, where each property is a key-value pair. Objects can contain any data type, including other objects.
Example:
javascript
let person = {
name: 'John',
age: 30,
isEmployed: true
};
Array: A special type of object used for storing ordered collections of values. Arrays are indexed, starting from 0.
Example:
javascript
let numbers = [1, 2, 3, 4, 5];
Function: Also a type of object, functions are reusable blocks of code that can be called with arguments and can return a value.
Example:
javascript
function greet(name) {
return `Hello, ${name}!`;
}
Date: Represents a single moment in time, down to the millisecond.
Example:
javascript
let now = new Date();
Type Conversion
JavaScript is dynamically typed, meaning that variables can hold values of any data type,
and the type can change during the execution of the program. There are two types of type conversion: implicit and explicit.
Implicit Conversion: Automatically performed by JavaScript.
Example:
javascript
let result = '5' + 2; // '52' (number 2 is
converted to
string '2')
Explicit Conversion: Manually performed by the developer.
Examples:
javascript
let num = Number('5'); // 5 (string '5' is
converted to
number 5) let str = String(123); // '123' (number 123 is
converted to string '123')
Type Checking
To check the type of a variable, you can use the typeof operator:
Example:
javascript
let age = 25;
console.log(typeof age); // 'number'
let name = 'Alice';
console.log(typeof name); // 'string'
Operators
1. Arithmetic Operators
Arithmetic operators are used to perform mathematical operations.
Addition (+): Adds two numbers.
javascript
let a = 5 + 3; // 8
Subtraction (-): Subtracts the second number from the first.
javascript
let b = 5 - 3; // 2
Multiplication (*): Multiplies two numbers.
javascript
let c = 5 * 3; // 15
Division (/): Divides the first number by the second.
javascript
let d = 5 / 2; // 2.5
Modulus (%): Returns the remainder of a division.
javascript
let e = 5 % 2; // 1
Exponentiation (**): Raises the first number to the power
of the second.
javascript
let f = 2 ** 3; // 8
Increment (++): Increases a number by one.
javascript
let g = 5;
g++; // 6
Decrement (--): Decreases a number by one.
javascript
let h = 5;
h--; // 4
2. Assignment Operators
Assignment operators are used to assign values to variables.
Assignment (=): Assigns the right operand to the left operand.
javascript
let i = 10;
Addition assignment (+=): Adds the right operand to the
left operand and assigns the result to the left operand.
javascript
let j = 10;
j += 5; // 15
Subtraction assignment (-=): Subtracts the right operand
from the left operand and assigns the result to the left operand.
javascript
let k = 10;
k -= 5; // 5
Multiplication assignment (*=): Multiplies the left operand by the
right operand and assigns the result to the left operand.
javascript
let l = 10;
l *= 2; // 20
Division assignment (/=): Divides the left operand by the
right operand and assigns the result to the left operand.
javascript
let m = 10;
m /= 2; // 5
Modulus assignment (%=): Performs modulus on the left operand by
the right operand and assigns the result to the left operand.
javascript
let n = 10;
n %= 3; // 1
Exponentiation assignment (**=): Raises the left operand to the power of
the right operand and assigns the result to the left operand.
javascript
let o = 2;
o **= 3; // 8
3. Comparison Operators
Comparison operators are used to compare two values and return a
boolean value (true or false).
Equal (==): Compares two values for equality (type conversion is allowed).
javascript
5 == "5"; // true
Strict equal (===): Compares two values for equality (no type conversion).
javascript
5 === "5"; // false
Not equal (!=): Compares two values for inequality (type conversion is allowed).
javascript
5 != "5"; // false
Strict not equal (!==): Compares two values for inequality
(no type conversion).
javascript
5 !== "5"; // true
Greater than (>): Checks if the left operand is greater than
the right operand.
javascript
5 > 3; // true
Greater than or equal (>=): Checks if the left operand is
greater than or equal to the right operand.
javascript
5 >= 5; // true
Less than (<): Checks if the left operand is less than the right operand.
javascript
3 < 5; // true
Less than or equal (<=): Checks if the left operand is less
than or equal to the right operand.
javascript
5 <= 5; // true
4. Logical Operators
Logical operators are used to combine multiple conditions.
Logical AND (&&): Returns true if both operands are true.
javascript
true && true; // true
Logical OR (||): Returns true if at least one of the operands
is true.
javascript
true || false; // true
Logical NOT (!): Inverts the boolean value.
javascript
!true; // false
5. Bitwise Operators
Bitwise operators perform bit-level operations on the operands.
Bitwise AND (&): Performs a bitwise AND.
javascript
5 & 1; // 1 (0101 & 0001)
Bitwise OR (|): Performs a bitwise OR.
javascript
5 | 1; // 5 (0101 | 0001)
Bitwise XOR (^): Performs a bitwise XOR.
javascript
5 ^ 1; // 4 (0101 ^ 0001)
Bitwise NOT (~): Performs a bitwise NOT.
javascript
~5; // -6 (~0101)
Left shift (<<): Shifts bits to the left.
javascript
5 << 1; // 10 (0101 << 1)
Right shift (>>): Shifts bits to the right.
javascript
5 >> 1; // 2 (0101 >> 1)
Unsigned right shift (>>>): Shifts bits to the right,
filling the left with zeros.
javascript
5 >>> 1; // 2 (0101 >>> 1)
6. String Operators
String operators are used to manipulate text strings.
Concatenation (+): Joins two or more strings.
javascript
let p = "Hello" + " " + "World"; // "Hello World"
Concatenation assignment (+=): Adds a string to another
string.
javascript
let q = "Hello";
q += " World"; // "Hello World"
7. Conditional (Ternary) Operator
The conditional (ternary) operator is a shorthand for the if-else statement.
javascript
let age = 18;
let canVote = (age >= 18) ? "Yes" : "No"; // "Yes"
8. Comma Operator
The comma operator allows you to evaluate multiple expressions
in a single statement, returning the value of the last expression.
javascript
let r = (2 + 3, 5 + 1); // 6
9. Type Operators
Type operators are used to check or change the type of a variable.
typeof: Returns the type of a variable.
javascript
typeof 42; // "number"
typeof "Hello"; // "string"
instanceof: Checks if an object is an instance of a particular
class or constructor function.
javascript
let arr = [];
arr instanceof Array; // true
10. Other Operators
in: Checks if a property exists in an object.
javascript
let obj = { name: "Alice" };
"name" in obj; // true
delete: Deletes a property from an object.
javascript
delete obj.name; // true
Like
Share
# Tags
Share
# Tags