JavaScript: Learn Coding Fundamentals
JavaScript is a powerful and versatile programming language that is essential to modern web development. Initially designed to make web pages interactive, it has grown to become a core component of the web, alongside HTML and CSS. Today, JavaScript not only runs in the browser but also on servers, mobile devices, and even robots.
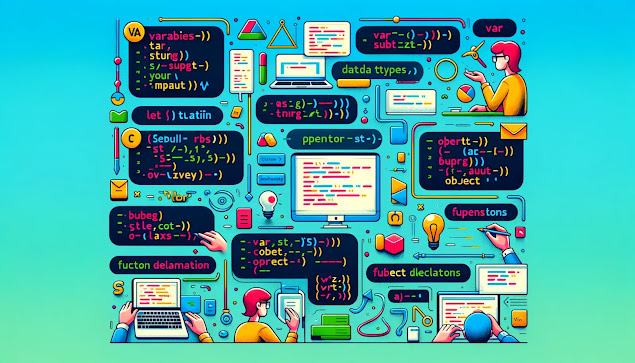
1. What is JavaScript?
JavaScript is a high-level, interpreted programming language known for its role in web development. Unlike languages that need to be compiled before running, JavaScript code can be written in a text file and run directly in a web browser or on a server using environments like Node.js.
2. Why Learn JavaScript?
- Ubiquity: JavaScript runs on nearly every device that has a web browser, making it one of the most universally deployed languages.
- Flexibility: JavaScript supports multiple programming paradigms, including object-oriented, imperative, and functional programming styles.
- Community and Resources: There is a vast community of developers who use JavaScript, providing a wealth of libraries, frameworks, and tools that enhance its capabilities and ease of use.
- Career Opportunities: Proficiency in JavaScript opens up numerous career opportunities in web development, mobile app development, and beyond.
3. Core Concepts of JavaScript
- Variables and Data Types: Learn about declaring variables with
var
,let
, andconst
, and explore data types like numbers, strings, booleans, objects, and arrays. - Operators: Understand how to use arithmetic, assignment, comparison, logical, and ternary operators to manipulate data and make decisions.
- Control Structures: Control the flow of your programs with if-else statements, switch cases, and loops (for, while, and do-while).
4. How JavaScript Works with HTML and CSS
JavaScript is often used in conjunction with HTML (to structure content) and CSS (to style content). It enhances user interfaces by dynamically modifying HTML and CSS, responding to user actions, and communicating with web servers for a seamless and dynamic user experience.
5. Basic Examples
- Hello World: Show how to use JavaScript to display a "Hello World" message on a web page.
- Interactive Button: Create a button that changes color when clicked, demonstrating how JavaScript responds to user events
6. Advanced JavaScript Concepts
After grasping the basics, it’s important to delve into more complex concepts that are vital for sophisticated web and application development.
- Functions: Understand first-class functions, function expressions, arrow functions, and higher-order functions. Learn how closures work and how to use them to maintain private variables within a function scope.
- Object-Oriented JavaScript: Explore objects in-depth, including constructors, prototype inheritance, and new ES6+ features like classes and modules.
- Asynchronous Programming: JavaScript's asynchronous programming capabilities are fundamental for handling operations like fetching data from a server. Learn about callbacks, promises, async/await, and handling asynchronous data flows elegantly.
7. JavaScript in the Ecosystem
Understanding how JavaScript interacts with the web ecosystem is crucial for full-stack development.
- DOM Manipulation: Learn how JavaScript interacts with the Document Object Model (DOM) to dynamically change content, style, and structure of web pages.
- Event Handling: Deep dive into how events are managed in JavaScript. Understand event propagation, default actions, and how to create interactive pages with event listeners.
- Web APIs: Explore how JavaScript uses various Web APIs for tasks like sending HTTP requests (Fetch API), storing data locally (LocalStorage, IndexedDB), and more.
8. Modern JavaScript Tools and Frameworks
As you advance, familiarizing yourself with modern tools and frameworks will greatly enhance your development skills.
- Node.js: Learn about this JavaScript runtime built on Chrome's V8 JavaScript engine that lets you run JavaScript on the server.
- Frameworks and Libraries: Get to know frameworks like React, Angular, and Vue.js which help in building client-side applications. Libraries like jQuery for simpler DOM manipulation tasks, and D3.js for data-driven visualizations.
- Build Tools and Transpilers: Understand the role of build tools like Webpack and Rollup, as well as transpilers like Babel, which allow developers to use next-generation JavaScript, today.
9. Practical Applications and Projects
Finally, applying what you've learned in real-world projects is the best way to solidify your knowledge.
- Single Page Application (SPA): Build a SPA using React or Angular that interacts with a backend API to perform CRUD operations.
- Real-time Chat Application: Create a real-time chat application using Node.js and WebSocket for real-time communication.
- E-commerce Site Features: Implement features like a shopping cart, product search, and user authentication
10. JavaScript Performance Optimization
Understanding performance optimization in JavaScript is crucial for developing efficient, fast-loading web applications.
- Efficient Code Writing: Learn the best practices for writing efficient JavaScript, including avoiding common pitfalls like excessive DOM manipulation or improper use of data structures.
- Memory Management: Explore JavaScript's garbage collection mechanism and strategies to manage memory effectively, including avoiding memory leaks.
- Performance Tools: Get acquainted with tools like Google's Lighthouse or Firefox’s Developer Tools to analyze and improve the performance of web applications.
11. Security in JavaScript
Security is a major concern in web development. Knowledge of common security threats and how to counteract them is essential for any developer.
- Common Vulnerabilities: Understand Cross-Site Scripting (XSS), Cross-Site Request Forgery (CSRF), and other common security vulnerabilities in web applications.
- Secure Coding Practices: Learn practices such as sanitizing user input, using HTTPS, and secure headers to protect against attacks.
- Using Security Libraries: Familiarize yourself with libraries and frameworks that help secure JavaScript code, such as OAuth for authentication or Helmet for securing HTTP headers.
12. Testing JavaScript
Testing is a critical part of the development process, ensuring that code is robust and behaves as expected.
- Unit Testing: Introduction to unit testing with frameworks like Jest or Mocha. Learn how to write test cases for JavaScript functions and components.
- Integration and Functional Testing: Learn how to set up and conduct integration tests that check if different parts of your application interact correctly. Explore functional testing to ensure the app works as intended for the end-user.
- End-to-End Testing: Understand tools like Cypress or Selenium that help developers automate browser testing for complex user interactions.
13. Advanced Projects and Use Cases
To truly master JavaScript, apply your skills to complex, real-world projects that challenge your understanding and creativity.
- Progressive Web App (PWA): Build a Progressive Web App that works offline using service workers and cache APIs. This project will involve both front-end and back-end JavaScript.
- Interactive Data Visualization: Create interactive data visualizations using JavaScript with libraries like Three.js for 3D visualizations or Chart.js for dynamic charts.
- Blockchain Basics: Implement a simple blockchain demonstration using Node.js to understand the basics of blockchain technology and how JavaScript can be utilized in emerging tech fields.
14. JavaScript with APIs and Asynchronous Programming
As JavaScript applications become more complex and interconnected with other web services, understanding how to work with APIs and handle asynchronous operations is crucial.
- Fetching Data: Teach how to use the
fetch
API in JavaScript to retrieve data from external APIs. This includes handling responses, parsing JSON, and error management. - Async/Await Syntax: Deepen knowledge of modern JavaScript asynchronous patterns, particularly the async/await syntax, which makes asynchronous code easier to write and read compared to traditional callback functions and promises.
- Working with RESTful Services: Explain the principles of RESTful services and how to interact with them using JavaScript, covering methods like GET, POST, PUT, and DELETE.
15. Scalable JavaScript Architectures
For JavaScript developers aiming to build large-scale applications, understanding architecture and design patterns is essential.
- Module Design Pattern: Introduce the module design pattern to encapsulate and manage the code base more effectively.
- MVC Framework: Dive into the Model-View-Controller (MVC) framework and how it can be implemented in JavaScript to create scalable and maintainable web applications.
- State Management: Explore state management techniques and tools like Redux or MobX, which are critical for managing state in large, complex applications with many dynamic elements and data flows.
16. Real-Time Applications with WebSockets
Real-time communication is a vital feature for modern web applications, such as chat applications, live notifications, and real-time data feeds.
- Understanding WebSockets: Explain the WebSocket protocol and how it allows for two-way interactive communication sessions between the user's browser and a server.
- Building a Real-Time Chat Application: Guide through building a basic real-time chat application using Node.js and Socket.IO, explaining the key concepts of real-time data transmission and reception.
- Advanced Use Cases: Discuss more advanced real-time applications such as live sports updates, stock tickers, and collaborative editing tools.
17. Contributing to Open Source
Engaging with the open-source community can greatly enhance a developer’s skills and visibility in the industry.
- Understanding Open Source: Discuss what open source is, the importance of open-source projects, and how they contribute to the tech community.
- How to Contribute: Guide on how to find suitable projects, understand their contribution guidelines, and make your first contribution.
- Best Practices: Cover best practices for open-source contributions, including writing readable code, documenting changes, and participating in community discussions.
18. Career Development with JavaScript
Finally, guide readers on how to leverage their JavaScript skills to advance their careers.
- Building a Portfolio: Tips on building a compelling portfolio that showcases a range of projects, from simple scripts to complex applications.
- Networking and Community Engagement: Advise on participating in coding meetups, conferences, and online forums to network with other developers and learn about job opportunities.
- Interview Preparation: Offer guidance on preparing for technical interviews, including common JavaScript questions, coding challenges, and problem-solving techniques.
Share
# Tags