A Starter Guide to Data Structures for AI and Machine Learning
Data structures are fundamental concepts in computer science that help organize and store data efficiently. In the context of AI and machine learning, understanding data structures is crucial because these fields often deal with large volumes of data that need to be processed and analyzed quickly. Here's a starter guide to some key data structures relevant to AI and machine learning:
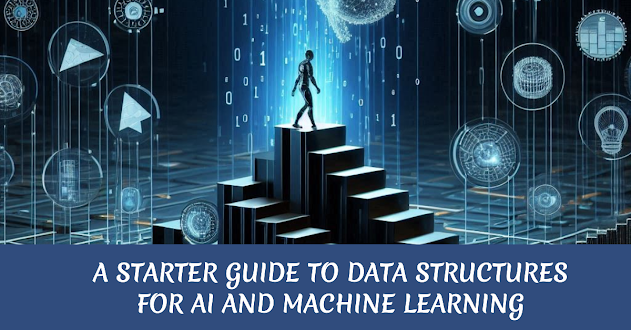
Arrays: Arrays are one of the simplest data structures, consisting of a collection of elements stored in contiguous memory locations. In AI and machine learning, arrays are often used to represent datasets, input features, or output predictions.
Lists: Lists are similar to arrays but more flexible because they can dynamically resize. In Python, for example, lists can grow or shrink as needed, making them useful for managing datasets of varying lengths.
Stacks: Stacks follow the Last In, First Out (LIFO) principle, where the last element added is the first one to be removed. Stacks are commonly used in algorithms for depth-first search and backtracking, which are relevant to certain AI and machine learning techniques.
Queues: Queues adhere to the First In, First Out (FIFO) principle, where the first element added is the first one to be removed. Queues are useful for implementing algorithms like breadth-first search, which is essential in various AI applications, such as pathfinding and network analysis.
Trees: Trees are hierarchical data structures consisting of nodes connected by edges. In AI and machine learning, decision trees are commonly used for classification tasks. Additionally, tree-based models like random forests and gradient boosting machines are widely employed for both classification and regression tasks.
Graphs: Graphs are collections of nodes (or vertices) connected by edges. They're used to model relationships between entities, making them invaluable in AI and machine learning for tasks like social network analysis, recommendation systems, and natural language processing.
Hash Tables: Hash tables are data structures that store key-value pairs, allowing for efficient retrieval of values based on their keys. They're useful for tasks like caching, indexing, and implementing associative arrays, which can be beneficial in various machine learning algorithms.
Heaps: Heaps are specialized trees that satisfy the heap property, where the value of each parent node is either greater than or equal to (max heap) or less than or equal to (min heap) the values of its children. Heaps are often used in priority queue implementations, which are useful in algorithms like Dijkstra's shortest path algorithm and A* search algorithm, both of which are important in AI for tasks like pathfinding and optimization.
Hashmaps: Hashmaps, also known as dictionaries or associative arrays, are data structures that store key-value pairs. They use a hash function to map keys to indices in an array, allowing for efficient lookup, insertion, and deletion of elements. Hashmaps are widely used in AI and machine learning for tasks like feature hashing in natural language processing and data preprocessing.
Trie: A trie, or prefix tree, is a tree data structure used to store a dynamic set of strings where each node represents a common prefix of its children. Tries are particularly useful in text processing tasks like autocomplete, spell checking, and searching, which are common in natural language processing applications of AI.
Sparse Matrices: In many AI and machine learning applications, datasets are high-dimensional but sparse, meaning most of the elements are zero. Sparse matrices are data structures optimized for storing and manipulating such datasets efficiently, reducing memory usage and computational overhead. Sparse matrices are commonly used in algorithms for tasks like collaborative filtering in recommendation systems and text mining in natural language processing.
Disjoint Set (Union-Find): Disjoint set data structure, also known as union-find data structure, is used to efficiently maintain a collection of disjoint (non-overlapping) sets and perform operations like union (combining two sets) and find (determining which set a particular element belongs to). Disjoint set data structure finds applications in various AI algorithms, including image segmentation, clustering, and connected component analysis.
Bloom Filters: Bloom filters are probabilistic data structures used to test whether an element is a member of a set. They offer a space-efficient way to represent large sets and provide constant-time lookup with a small probability of false positives. Bloom filters find applications in various AI tasks, such as data deduplication, web caching, and network intrusion detection.
Skip Lists: Skip lists are probabilistic data structures that provide an efficient alternative to balanced trees for maintaining a sorted sequence of elements. They allow for fast search, insertion, and deletion operations with average-case logarithmic time complexity, making them suitable for implementing ordered data structures in AI applications like indexing in databases and search engines.
KD-Trees: KD-trees, or k-dimensional trees, are space-partitioning data structures used for organizing points in a k-dimensional space. They facilitate efficient nearest neighbor search and range query operations, making them valuable in machine learning algorithms such as k-nearest neighbors (KNN) classification and clustering.
Suffix Arrays and Suffix Trees: Suffix arrays and suffix trees are data structures used to store all suffixes of a given string in lexicographical order. They enable efficient substring search and various string manipulation operations, which are essential in natural language processing tasks like text indexing, pattern matching, and sequence alignment.
Probabilistic Data Structures: Probabilistic data structures are specialized data structures that use probabilistic techniques to provide approximate solutions to certain problems with reduced memory requirements and processing time. Examples include Count-Min Sketch for frequency estimation, HyperLogLog for cardinality estimation, and Bloom Filters for set membership testing, all of which have applications in AI tasks like stream processing, large-scale analytics, and distributed systems.
Self-Balancing Trees: Self-balancing trees, such as AVL trees, red-black trees, and B-trees, are tree data structures that automatically adjust their shape to maintain balance, ensuring efficient insertion, deletion, and search operations even in the presence of dynamic data. They are widely used in database systems, indexing structures, and search algorithms employed in AI applications like information retrieval, data mining, and knowledge discovery.
Spatial Data Structures: These data structures are designed to efficiently store and query spatial data, such as points, lines, and polygons, in multidimensional space. Examples include quad trees, octrees, and R-trees, which are commonly used in geographic information systems (GIS), computer graphics, and spatial indexing for tasks like nearest neighbor search, range queries, and spatial clustering.
Persistent Data Structures: Persistent data structures are immutable data structures that preserve previous versions of themselves when modified, enabling efficient time-traveling operations and facilitating concurrency and parallelism. While not as common in traditional machine learning applications, persistent data structures find applications in functional programming, concurrent data structures, and version control systems, which are increasingly relevant in modern AI systems.
Graph Neural Networks (GNNs) Data Structures: With the rise of graph neural networks, specialized data structures and algorithms have been developed to efficiently represent and process graph-structured data. Graph data structures, such as adjacency lists and adjacency matrices, along with graph algorithms like breadth-first search (BFS) and depth-first search (DFS), form the foundation for GNNs, enabling tasks like node classification, link prediction, and graph-level prediction in diverse applications including social network analysis, recommendation systems, and drug discovery.
Temporal Data Structures: Temporal data structures are designed to handle time-series data, where each data point is associated with a timestamp. These structures enable efficient storage, indexing, and retrieval of temporal data, supporting tasks like trend analysis, anomaly detection, and forecasting in various domains including finance, healthcare, and environmental monitoring. Examples include time series databases, sliding window data structures, and temporal indexes.
Hypergraph Data Structures: Hypergraphs extend the concept of graphs by allowing hyperedges to connect multiple nodes simultaneously, enabling the representation of complex relationships among entities. Hypergraph data structures and algorithms are increasingly employed in AI applications requiring higher-order interactions and dependencies, such as hypergraph-based clustering, hypergraph-based learning, and knowledge representation in semantic web and knowledge graphs.
Probabilistic Graphical Models (PGMs) Data Structures: Probabilistic graphical models represent probabilistic relationships among random variables using graph-based structures, such as Bayesian networks (directed graphs) and Markov random fields (undirected graphs). Efficient data structures and algorithms for inference, learning, and inference, such as message passing algorithms and junction trees, are essential for performing probabilistic reasoning, inference, and learning tasks in AI applications like probabilistic reasoning, pattern recognition, and decision making.
- Suffix Trees: Suffix trees are a powerful data structure used to efficiently store and process strings or sequences. They represent all suffixes of a given string in a compressed trie-like structure, enabling fast substring search, longest common substring computation, and various string manipulation tasks. Suffix trees find applications in diverse areas of AI and machine learning, including bioinformatics (genome sequence analysis, sequence alignment), text processing (pattern matching, plagiarism detection), and data compression (Burrows-Wheeler Transform).
Share
# Tags