Exploring Python's itertools Module: Unlocking the Power of Iterators
In the realm of Python programming, iterators play a crucial role in facilitating efficient and memory-friendly iteration over data structures. The `itertools` module in Python is a powerful toolkit that offers a plethora of functions for creating and manipulating iterators. In this article, we'll delve into the depths of Python's `itertools` module to unlock its full potential and understand how it can streamline various iterative tasks.
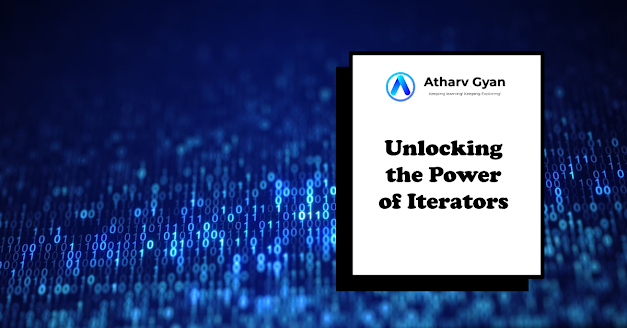
Understanding Iterators:
Before we embark on exploring the `itertools` module, let's briefly recap what iterators are in Python. An iterator is an object that represents a stream of data. It enables sequential access to elements of a collection or a sequence without exposing the underlying implementation details. Iterators are used extensively in Python for looping constructs, such as `for` loops, and are an essential component of many built-in functions and modules.
Introduction to itertools Module:
The `itertools` module is a part of Python's standard library and provides a collection of functions for creating iterators for efficient looping and data manipulation. It offers a wide range of tools for working with iterators, including functions for permutations, combinations, cycling, and more. By leveraging the functions provided by `itertools`, developers can write concise and expressive code for handling complex iteration tasks.
Key Functions in itertools:
1.Permutations and Combinations: The `itertools.permutations()` and `itertools.combinations()` functions allow us to generate all possible permutations and combinations of elements from a given iterable. These functions are particularly useful for tasks involving combinatorial problems, such as generating permutations of a set of characters or finding combinations of elements that satisfy certain criteria.
2. Infinite Iterators: `itertools` offers several functions for creating infinite iterators, such as `itertools.count()` and `itertools.cycle()`. These iterators can be used to generate an infinite sequence of numbers or cycle through a finite sequence indefinitely, providing a convenient way to work with unbounded data streams or implement looping constructs with no predetermined endpoint.
3. Chaining and Grouping: The `itertools.chain()` function allows us to chain together multiple iterators into a single iterable sequence. This can be useful for concatenating sequences or combining data from different sources. Additionally, `itertools.groupby()` enables us to group elements of an iterable based on a common key function, facilitating the segmentation and aggregation of data in a flexible and efficient manner.
Practical Examples:
Let's illustrate the usage of `itertools` with a couple of practical examples:
1. Generating Permutations:
```python
import itertools
# Generate all permutations of 'ABC'
perms = itertools.permutations('ABC')
print(list(perms)) # Output: [('A', 'B', 'C'), ('A', 'C', 'B'), ('B', 'A', 'C'), ...]
```
2. Creating an Infinite Counter:
```python
import itertools
# Create an infinite counter starting from 1
counter = itertools.count(start=1)
for _ in range(5):
print(next(counter)) # Output: 1, 2, 3, 4, 5
```
3. Combining Multiple Iterators with Chain:
```python
import itertools
# Define multiple iterators
iter1 = iter([1, 2, 3])
iter2 = iter(['a', 'b', 'c'])
# Chain the iterators together
chained_iter = itertools.chain(iter1, iter2)
# Iterate over the chained iterator
for item in chained_iter:
print(item) # Output: 1, 2, 3, 'a', 'b', 'c'
```
4. Grouping Elements by Key:
```python
import itertools
# Define a list of tuples with (name, score) pairs
students = [
('Alice', 85),
('Bob', 75),
('Alice', 90),
('Bob', 80),
('Charlie', 95)
]
# Group students by name
grouped_students = itertools.groupby(students, key=lambda x: x[0])
# Print average score for each student
for name, group in grouped_students:
scores = [score for _, score in group]
avg_score = sum(scores) / len(scores)
print(f"{name}: Average Score = {avg_score}")
```
Output:
```
Alice: Average Score = 87.5
Bob: Average Score = 77.5
Charlie: Average Score = 95.0
```
5. Creating Cartesian Products:
```python
import itertools
# Define two sets
set1 = {'A', 'B', 'C'}
set2 = {'X', 'Y'}
# Compute the Cartesian product of the sets
cartesian_product = itertools.product(set1, set2)
# Print the Cartesian product
for item in cartesian_product:
print(item) # Output: ('A', 'X'), ('A', 'Y'), ('B', 'X'), ('B', 'Y'), ('C', 'X'), ('C', 'Y')
```
6. Generating Combinations with Replacement:
```python
import itertools
# Generate combinations with replacement for a sequence of numbers
combinations_with_replacement = itertools.combinations_with_replacement(range(1, 4), 2)
# Print the combinations
for combination in combinations_with_replacement:
print(combination) # Output: (1, 1), (1, 2), (1, 3), (2, 2), (2, 3), (3, 3)
```
7. Iterating over Infinite Cycle:
```python
import itertools
# Define a list of colors
colors = ['red', 'green', 'blue']
# Create an infinite cycle of colors
color_cycle = itertools.cycle(colors)
# Print the first 10 colors in the cycle
for _ in range(10):
print(next(color_cycle)) # Output: red, green, blue, red, green, blue, ...
```
8. Creating Repeatable Iterators:
```python
import itertools
# Repeat a value infinitely
repeated_iterator = itertools.repeat('Hello', times=3)
# Print the repeated values
for item in repeated_iterator:
print(item) # Output: Hello, Hello, Hello
```
9. Grouping Data into Fixed-Length Tuples:
```python
import itertools
# Define a list of numbers
numbers = [1, 2, 3, 4, 5, 6]
# Group the numbers into fixed-length tuples
grouped_tuples = itertools.zip_longest(*[iter(numbers)]*3, fillvalue=None)
# Print the grouped tuples
for group in grouped_tuples:
print(group) # Output: (1, 2, 3), (4, 5, 6)
```
10. Calculating Running Sums:
```python
import itertools
# Define a list of numbers
numbers = [10, 20, 30, 40, 50]
# Calculate running sums
running_sums = itertools.accumulate(numbers)
# Print the running sums
for sum_ in running_sums:
print(sum_) # Output: 10, 30, 60, 100, 150
```
11. Generating Infinite Iterators with Accumulated Values:
```python
import itertools
# Generate an infinite iterator with accumulated values
accumulated_values = itertools.accumulate(itertools.count())
# Print the accumulated values up to a certain limit
for value in itertools.islice(accumulated_values, 5):
print(value) # Output: 0, 1, 3, 6, 10
```
This example demonstrates how to use `itertools.accumulate()` with `itertools.count()` to create an infinite iterator that produces accumulated values.
12. Pairwise Iteration:
```python
import itertools
# Define a list of numbers
numbers = [1, 2, 3, 4, 5]
# Pairwise iteration over adjacent elements
pairs = zip(numbers, numbers[1:])
# Print the pairwise combinations
for pair in pairs:
print(pair) # Output: (1, 2), (2, 3), (3, 4), (4, 5)
```
Here, `zip()` is used with list slicing to iterate over adjacent pairs of elements in a list.
13. Generating Unique Combinations:
```python
import itertools
# Define a list of colors
colors = ['red', 'green', 'blue']
# Generate unique combinations of two colors
unique_combinations = itertools.combinations(colors, 2)
# Print the unique combinations
for combination in unique_combinations:
print(combination) # Output: ('red', 'green'), ('red', 'blue'), ('green', 'blue')
```
This example uses `itertools.combinations()` to generate all unique combinations of two colors from a list.
14. Grouping Adjacent Elements by a Predicate:
```python
import itertools
# Define a list of numbers
numbers = [1, 2, 3, 4, 5, 6, 7, 8, 9]
# Group adjacent even and odd numbers
grouped_numbers = itertools.groupby(numbers, key=lambda x: x % 2 == 0)
# Print the grouped numbers
for is_even, group in grouped_numbers:
print(f"{'Even' if is_even else 'Odd'} numbers:", list(group))
```
This example utilizes `itertools.groupby()` with a custom key function to group adjacent elements based on whether they are even or odd.
15. Generating Infinite Iterators with Repeating Patterns:
```python
import itertools
# Define a repeating pattern of values
pattern = itertools.cycle([1, 2, 3])
# Generate an infinite iterator with repeating pattern
repeating_pattern = itertools.islice(pattern, 10)
# Print the repeating pattern
print(list(repeating_pattern)) # Output: [1, 2, 3, 1, 2, 3, 1, 2, 3, 1]
```
This example uses `itertools.cycle()` to create an infinite iterator with a repeating pattern of values, which is then sliced to generate a finite sequence.
16. Combining Iterators with a Custom Function:
```python
import itertools
# Define two iterators with numbers
iter1 = iter([1, 2, 3])
iter2 = iter([4, 5, 6])
# Combine iterators using a custom function
combined_iter = itertools.starmap(lambda x, y: x * y, zip(iter1, iter2))
# Print the combined values
print(list(combined_iter)) # Output: [4, 10, 18]
```
Here, `itertools.starmap()` is used with `zip()` to combine values from two iterators using a custom function.
17. Generating Iterators with Infinite Ranges:
```python
import itertools
# Generate an infinite iterator with an increasing range
increasing_range = itertools.count(start=1, step=2)
# Print the first 5 elements of the increasing range
print(list(itertools.islice(increasing_range, 5))) # Output: [1, 3, 5, 7, 9]
```
This example demonstrates how to create an infinite iterator with an increasing range of odd numbers using `itertools.count()`.
18. Combining Multiple Iterators into a Single Iterator:
```python
import itertools
# Define three iterators with numbers
iter1 = iter([1, 2, 3])
iter2 = iter([4, 5, 6])
iter3 = iter([7, 8, 9])
# Combine multiple iterators into a single iterator
combined_iter = itertools.chain(iter1, iter2, iter3)
# Print the combined values
print(list(combined_iter)) # Output: [1, 2, 3, 4, 5, 6, 7, 8, 9]
```
Here, `itertools.chain()` is used to combine multiple iterators into a single iterator that yields values from each input iterator sequentially.
19. Grouping Data by Frequency:
```python
import itertools
# Define a list of elements
elements = [1, 1, 2, 2, 2, 3, 3, 3, 3]
# Group elements by frequency
grouped_elements = itertools.groupby(elements)
# Print the frequency of each element
for key, group in grouped_elements:
frequency = len(list(group))
print(f"Element {key}: Frequency = {frequency}")
```
This example uses `itertools.groupby()` to group elements by their frequency, providing insight into how many times each element appears consecutively.
Like
Share
# Tags
Share
# Tags